1669. Merge In Between Linked Lists
You are given two linked lists: list1 and list2 of sizes n and m respectively.
Remove list1’s nodes from the ath node to the bth node, and put list2 in their place.
The blue edges and nodes in the following figure indicate the result:
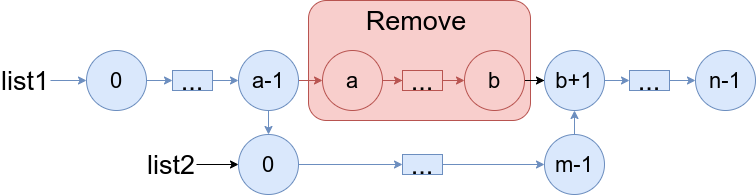
Build the result list and return its head.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
class Solution { ListNode* getNthNode(ListNode* node, int n) { ListNode* runner = node; while(n--) { runner = runner->next; } return runner; } public: ListNode* mergeInBetween(ListNode* list1, int a, int b, ListNode* list2) { ListNode* tail = list2; while(tail->next) { tail = tail->next; } tail->next = getNthNode(list1, b + 1); getNthNode(list1, a - 1)->next = list2; return list1; } };
|