Builder Pattern
빌더 패턴은 객체를 생성하는 방법과 표현하는 방법을 정의하는 클래스를 나누어 깔끔하고 유연하게 객체를 생성할 수 있는 패턴이다.
활용성
- 각 인자가 어떤 의미인지 알고싶을 때
- 여러개의 생성자를 생성하고 싶지 않을 때
빌더 패턴 사용에 따른 결과
생성과 표현에 필요한 코드 분리
빌더 패턴을 사용하면 복합 객체를 생성하고 복합 객체의 내부 표현 방법을 별도의 모듈로 정의할 수 있다. 사용자는 제품의 내부 구조를 정의한 클래스는 모른채 빌더와 상호작용을 통해 필요한 복합 객체를 생성해낸다.
유연한 객체의 생성
빌더 패턴을 사용하면 다양한 생성자를 정의하지 않고도 유연하게 객체를 생성할 수 있다. 또한 각 멤버 변수 할당시 의미가 명확해진다.
빌더
구조
빌더의 구조는 다음과 같다.
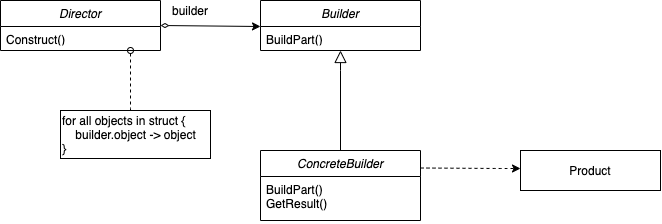
C++ 구현
1 2 3 4 5 6 7 8 9 10
| void BuilderPattern::build() { Pizza* pepperoniPizza = Pizza::Builder::builder() .setDough("Flour").setSauce("Tomato").setTopping("Pepperoni").build(); Pizza* pineapplePizza = Pizza::Builder::builder() .setDough("Flour").setTopping("PineApple").build(); pepperoniPizza->showPizzaInfo(); pineapplePizza->showPizzaInfo();
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73
| class Pizza { std::string topping; std::string dough; std::string sauce;
public: static class Builder { private: std::string topping; std::string dough; std::string sauce;
public: static Builder builder() { Builder builder; return builder; }
Builder setTopping(std::string topping) { this->topping = std::move(topping); return *this; }
Builder setDough(std::string dough) { this->dough = std::move(dough); return *this; }
Builder setSauce(std::string sauce) { this->sauce = std::move(sauce); return *this; }
std::string getTopping() { return topping; }
std::string getDough() { return dough; }
std::string getSauce() { return sauce; }
Pizza* build() { return new Pizza(*this); } };
public: Pizza(Builder builder) : topping(builder.getTopping()), dough(builder.getDough()), sauce(builder.getSauce()) {}
void showPizzaInfo() { std::cout<<"Dough : "<<dough<<std::endl; std::cout<<"Sauce : "<<sauce<<std::endl; std::cout<<"Topping : "<<topping<<std::endl; } };
|
java 구현
1 2 3 4 5 6 7 8 9
| public class Client { static public void main(String[] args) { Pizza pepperoniPizza = new Pizza.Builder().dough("Flour").sauce("Tomato") .topping("Pepperoni").build(); Pizza pineapplePizza = new Pizza.Builder().dough("Flour").topping("PineApple").build(); System.out.println(pepperoniPizza.toString()); System.out.println(pineapplePizza.toString()); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| public class Pizza { private String topping; private String dough; private String sauce;
public static class Builder { private String topping; private String dough; private String sauce;
Builder topping(String topping) { this.topping = topping; return this; }
Builder dough(String dough) { this.dough = dough; return this; }
Builder sauce(String sauce) { this.sauce = sauce; return this; }
Pizza build() { return new Pizza(this); } }
private Pizza(Builder builder) { this.topping = builder.topping; this.dough = builder.dough; this.sauce = builder.sauce; }
@Override public String toString() { StringBuilder sb = new StringBuilder(); sb.append("Dough : "); sb.append(dough); sb.append("\n"); sb.append("Sauce : "); sb.append(sauce); sb.append("\n"); sb.append("Topping : "); sb.append(topping); sb.append("\n"); return sb.toString(); } }
|