Facade Pattern
퍼사드 패턴은 한 서브시스템 내의 인터페이스 집합에 대한 획일화된 하나의 인터페이스를 제공하는 패턴으로 서브시스템을 사용하기 쉽도록 상위 수준의 인터페이스를 제공한다.
활용성
- 복잡한 서브시스템에 대한 단순한 인터페이스 제공이 필요할 때
- 추상 개념에 대한 구현 클래스와 사용자 사이에 너무 많은 종속성이 존재할 때
- 서브시스템을 계층화시킬 때
퍼사드 패턴 사용에 따른 결과
서브시스템의 구성요소 보호
서브시스템의 구성요소를 보호함으로 써 사용자가 다루어야할 객체의 수가 줄어들며 서브시스템을 쉽게 사용할 수 있다.
서브시스템과 사용자 코드간 결합도 저하
사용자 코드에서 서브시스템을 직접 사용하면 결합도가 높아지기 마련이다. 퍼사드 패턴을 통해 획일화된 인터페이스를 제공함으로써 결합도를 낮출 수 있다.
서브시스템 사용자 코드에서 호출 가능
서브시스템을 사용자 코드에서 직접 사용하는것을 막지는 않는다. 그렇기 때문에 사용자가 퍼사드를 사용할지 서브시스템을 사용할지 결정권을 부여할 수 있다.
퍼사드
구조
퍼사드의 구조는 다음과 같다.
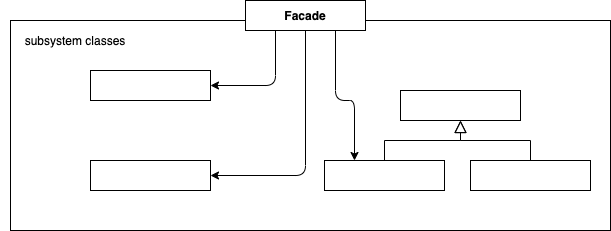
Facade Pattern Diagram
C++ 구현
c++1 2 3 4 5 6
| void FacadePattern::runRobot() { Robot* robot = new Robot(); robot->runRobot(); robot->offRobot(); }
|
c++1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Robot { RobotEye* robotEye; RobotArm* robotArm; RobotLeg* robotLeg; public: Robot() { this->robotEye = new RobotEye(); this->robotArm = new RobotArm(); this->robotLeg = new RobotLeg(); }
void runRobot() { robotEye->run(); robotArm->run(); robotLeg->run(); }
void offRobot() { robotEye->off(); robotArm->off(); robotLeg->off(); } };
|
c++1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| class RobotEye { public: RobotEye() {}
void run() { std::cout<<"Robot Eye Blinking"<<std::endl; }
void off() { std::cout<<"Robot Eye off"<<std::endl; } };
class RobotArm { public: RobotArm() {}
void run() { std::cout<<"Robot Arm Shaking"<<std::endl; }
void off() { std::cout<<"Robot Arm off"<<std::endl; } };
class RobotLeg { public: RobotLeg() {}
void run() { std::cout<<"Robot Leg Walking"<<std::endl; }
void off() { std::cout<<"Robot Leg off"<<std::endl; } };
|
java 구현
java1 2 3 4 5 6 7
| public class Client { public static void main(String[] args) { Robot robot = new Robot(); robot.runRobot(); robot.offRobot(); } }
|
java1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| public class Robot { private RobotEye robotEye; private RobotArm robotArm; private RobotLeg robotLeg;
public Robot() { this.robotEye = new RobotEye(); this.robotArm = new RobotArm(); this.robotLeg = new RobotLeg(); }
public void runRobot() { this.robotEye.run(); this.robotArm.run(); this.robotLeg.run(); }
public void offRobot() { this.robotEye.off(); this.robotArm.off(); this.robotLeg.off(); } }
|
java1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| public class RobotEye { public void run() { System.out.println("Robot Eye Blinking"); }
public void off() { System.out.println("Robot Eye off"); } }
public class RobotArm { public void run() { System.out.println("Robot Arm Shaking"); }
public void off() { System.out.println("Robot Arm off"); } }
public class RobotLeg { public void run() { System.out.println("Robot Leg Walking"); }
public void off() { System.out.println("Robot Leg off"); } }
|